Side by Side and Stacked Bar Charts
In this class, We discuss Side by Side and Stacked Bar Charts.
For Complete YouTube Video: Click Here
The reader should have prior knowledge of constructing bar charts. Click here.
Side by Side Bar Charts
First, we take an example to construct side-by-side bar charts.
We are considering the superstore data set. In our previous classes, we discussed the data set.
Based on the assumption reader have prior knowledge of the data set—the explanation given in this class.
To construct the side-by-side bar charts, we take the total sale and furniture sales according to region.
We provide the code to take the total and furniture sale below.
import pandas as pd
df=pd.read_excel('sampledata.xls',sheet_name='Orders')
print(df.head())
# Taking the sum of the sale group wise
temp=pd.DataFrame(df.groupby(['Region'])['Sales'].agg('sum'))
print(temp)
x=temp.index
y=temp['Sales'].values# y shows sum of sale
print(x)
# code to two level grouping
temp=pd.DataFrame(df.groupby(['Region','Category'])['Sales'].agg('sum'))
print(temp)
x1=temp.index
print(x1)
# code to take Furniture sum of sale group wise
temp1=temp.xs('Furniture', level='Category')
print(temp1)
y1=temp1['Sales'].values# y1 gives sum of furniture sale group wise
print(y1)
Below is the code to construct side-by-side bar charts.
# Side by Side Bar Chart
import matplotlib.pyplot as plt
import numpy as np
x1=np.arange(len(x))
z=plt.figure(figsize=(18,5))
plt.bar(x1, y, color ='blue',width = 0.2)
plt.bar(x1+0.2,y1,color='orange',width=0.2)
plt.xlabel("Region")
plt.ylabel("Sum of sale")
plt.title("Region Wise Total Sale")
plt.legend(['Total','Furniture'],loc=0)
plt.show()
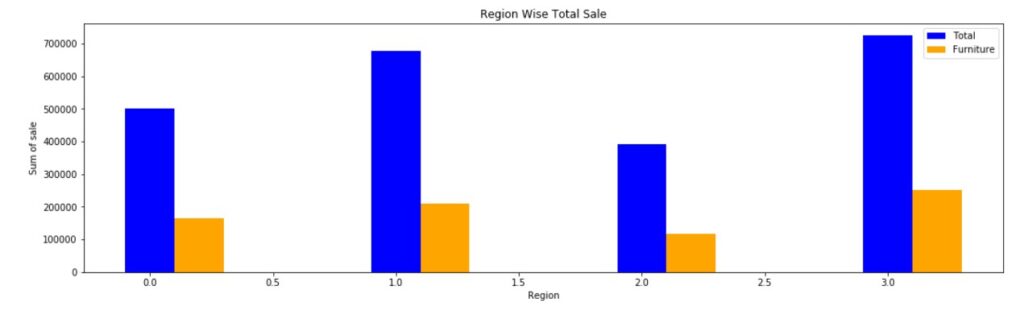
In the above code, we are using arrange function from numpy module.
The variable x contains the regions. there are four regions.
len(x) will give four value.
The arrange function will generate values 0,1,2,3.
We are using the bar function two times. The x-axis value in the second function is x1+0.2.
The bar is placed at position x1+0.2.
To do this addition, we considered the x-axis values numeric.
According to our requirement x-axis should have region values. How to place region values?
We use xticks to place region values.
Side by Side Bar Charts by placing xticks
The code to place the x-axis region values is given below.
# Side by Side by placing xticks
import matplotlib.pyplot as plt
import numpy as np
x1=np.arange(len(x))
z=plt.figure(figsize=(18,5))
plt.bar(x1, y, color ='blue',width = 0.2)
plt.bar(x1+0.2,y1,color='orange',width=0.2)
plt.xlabel("Region")
plt.ylabel("Sum of sale")
plt.title("Region Wise Total Sale")
plt.xticks(x1,x,horizontalalignment="center")
plt.legend(['Total','Furniture'],loc=0)
plt.show()
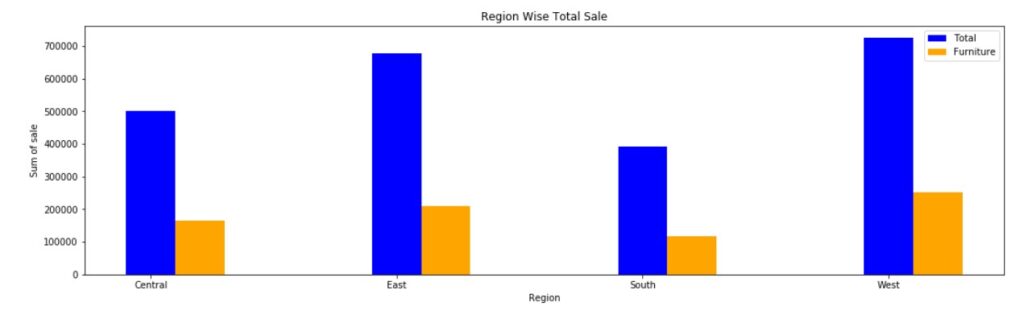
xticks Rotation: The code to rotate the xticks is given below.
# xticks Rotation
import matplotlib.pyplot as plt
import numpy as np
x1=np.arange(len(x))
z=plt.figure(figsize=(18,5))
plt.bar(x1, y, color ='blue',width = 0.2)
plt.bar(x1+0.2,y1,color='orange',width=0.2)
plt.xlabel("Region")
plt.ylabel("Sum of sale")
plt.title("Region Wise Total Sale")
plt.xticks(x1,x,rotation=90)
plt.legend(['Total','Furniture'],loc=0)
plt.show()
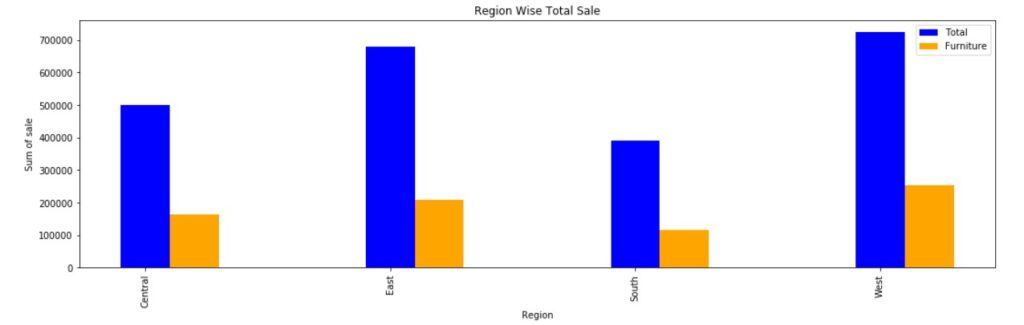
We use the method xticks from matplotlib.pyplot. And rotate parameter is used.
Stacked Bar Charts One on Another
The example we used in side-by-side bar charts is used here.
The bars are shown one above the other.
If we do not mention the position on the x-axis, we get the stacked bars.
The example program is given below.
# stacked Bar Chart one on another
import matplotlib.pyplot as plt
z=plt.figure(figsize=(18,5))
plt.bar(x, y, color ='blue',width = 0.2)
plt.bar(x,y1,color='orange',width=0.2)
plt.xlabel("Region")
plt.ylabel("Sum of sale")
plt.title("Region Wise Total Sale")
plt.legend(['Total','Furniture'],loc=0)
plt.show()
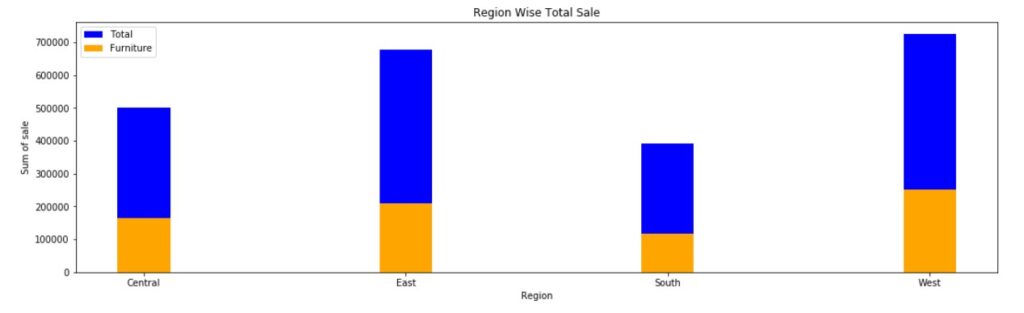
Stacked Bar Charts one above the other
In this example, we are considering the total sale of furniture, Office Supplies, and Technology.
The bars are shown one above the other.
To show the bars one above the other, we use the parameter bottom.
The second bar function bottom parameter takes argument y1.
The argument y1 tells the bar should start above y1.
Y1 is the bar top taken in the first bar function.
The third bar function takes the argument bottom as y1+y2.
Using this option, we construct stacked bar charts, one above the other.
The code to show stacked bar charts is given below.
# Stacked Bar Charts One above the Other
# code to take sum of sale all categories group wise
temp1=temp.xs('Furniture', level='Category')
temp2=temp.xs('Office Supplies', level='Category')
temp3=temp.xs('Technology', level='Category')
y1=temp1['Sales'].values
y2=temp2['Sales'].values
y3=temp3['Sales'].values
import matplotlib.pyplot as plt
import numpy as np
x1=np.arange(len(x))
z=plt.figure(figsize=(18,5))
plt.bar(x1, y1, color ='blue',width = 0.2)
plt.bar(x1,y2,bottom=y1,color='orange',width=0.2)
plt.bar(x1,y3,bottom=y1+y2,color='red',width=0.2)
plt.xlabel("Region")
plt.ylabel("Sum of sale")
plt.title("Region Wise Total Sale")
plt.legend(['Furniture','office','Technology'],loc=0)
plt.show()
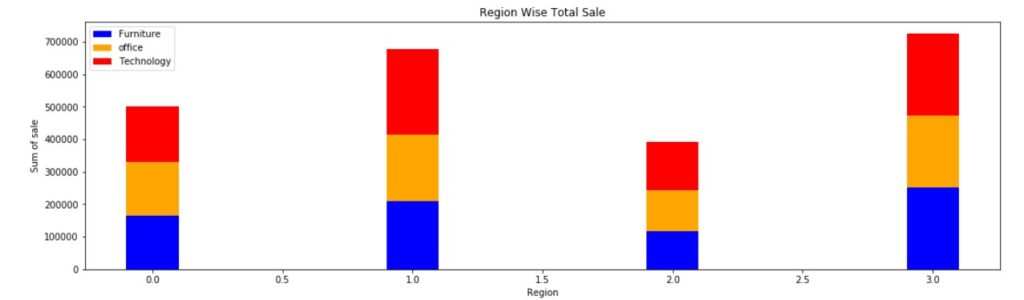