Bitwise Operators in C
For Complete YouTube Video: Click Here
In this class, we will try to understand Bitwise Operators in C.
In the previous classes, we have cover Arithmetic, Assignment, and Increment and Decrement Operators.
The Bitwise Operators are used to perform bit-level operations in a program.
Bitwise operators are binary operators.
Table of Contents
Types of Bitwise operators
The image below shows all the bitwise operators.
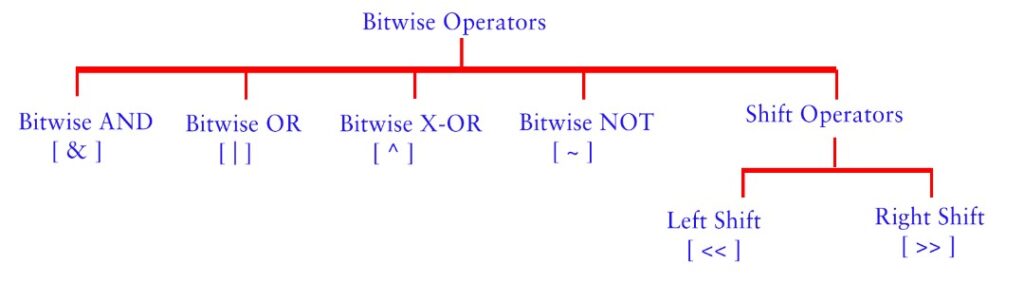
The different types of bitwise operators are
- Bitwise AND [ & ]
- Bitwise OR [ | ]
- Bitwise X-OR [ ^ ]
- Bitwise NOT [ ~ ]
- Left Shift Operator [ << ]
- Right Shift operator [ >> ]
In this class, we will cover the first four operators.
In the next class, we will cover “left shift” and “right shift” operators.
We will try to understand the concept of bitwise operators by using the program given below.
int main()
{
int a = 3, b = 5;
printf("Bitwise a&b is %d\n", a&b);
printf("Bitwise a|b is %d\n", a|b);
printf("Bitwise a^b is %d\n", a^b);
printf("Bitwise ~a is %d\n", a=~a);
return 0;
}
In the above program, the variable a is stored with three and b is stored with five.
The binary value of the decimal number 3 in eight-bit format is 00000011.
The binary value of the decimal number 5 in an eight-bit format is 00000101.
Use this tool to convert the decimal number to binary number.
Bitwise Operators AND [ & ]
A bitwise AND [ & ] operator produces an output of 1 if and only if both the operands are 1.
If any one of the operands is zero, it will produce an output of zero.
The image below shows how the bitwise AND operations are performed.
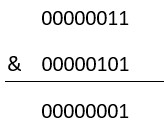
The first printf() function will print the decimal value of the result after the bitwise AND operation.
Bitwise Operators OR [ | ]
A bitwise OR [ | ] operator produces one if any operands are one or both the operands are 1.
If both the operands are zero, it will produce an output of zero.
The image below shows how the bitwise OR operations are performed.
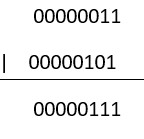
The second printf() function will print the decimal value of the result after the bitwise OR operation.
Bitwise Operators X-OR [ ^ ]
A bitwise X-OR [ ^ ] operator produces one if any operands are one.
If both the operands are zero or both the operands are one, it will produce zero.
The image below shows how the bitwise X-OR operations are performed.
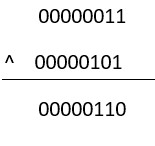
in C
The third printf() function will print the decimal value of the result after the bitwise X-OR operation.
Bitwise Operators NOT [ ~ ]
Bitwise NOT is a unary operator.
The bitwise NOT complement each bit of the binary value.
Bitwise NOT will convert binary 0 to 1 and binary 1 to 0.
The image below shows how the bitwise NOT operations are performed.
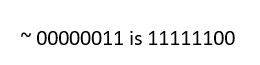
The fourth printf() function will print the decimal value of the result after the bitwise NOT operation.
The output of the above program is as shown below.
Output:
Bitwise a&b is 1
Bitwise a|b is 7
Bitwise a^b is 6
Bitwise ~a is -4