Pointer Arithmetic in C
In this class, we will try to understand Pointer Arithmetic in C.
In our previous class, we have seen the basics of pointers and arrays.
Table of Contents
Pointer Arithmetic
C supports some arithmetic operations on pointers
- Adding or subtracting an integer from a pointer
- Subtracting One Pointer from another
- Comparing Pointers
Adding or subtracting an integer from a pointer
We will try to understand the concept of adding and subtracting an integer from a pointer using the example shown below.
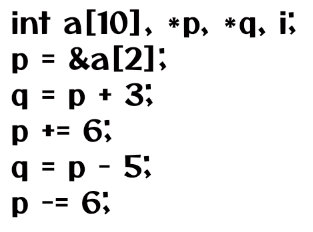
We will try to visualize the concepts line by line with images.
After executing the first line of code, an array of ten elements, pointers p and q, and a variable ‘i’ will get created, as shown in the image below.
Assume the integer is occupying two bytes of space.
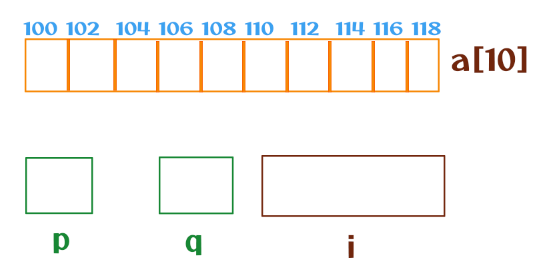
In the second line of code pointer, variable p will be pointing to ‘a[2]’ memory location.
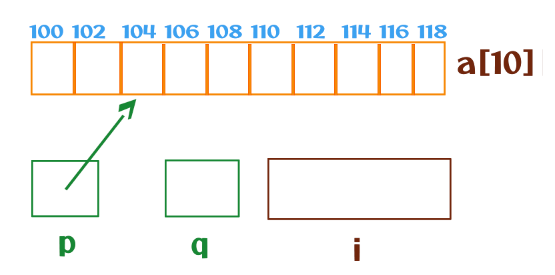
In the third line of code, we see the use of adding an integer to the pointer.
What happens when we add an integer to a pointer variable?
Will it work the same way as the general arithmetic’s.
No, here, the arithmetic’s are a bit different from the normal arithmetic’s.
The line of code is q = p + 3.
The value stored in p is 104. By adding 3 to 104, we have to get 107.
But in pointer arithmetic’s, we move three elements away from the 104 memory location.
The arithmetic’s followed is Address + (Number to be added * Size of the element).
Based on the above formula the value is 104 + (3 * 2) = 110.
The q will point to 110 memory locations which is the starting address of the sixth element of an array, as shown in the image below.
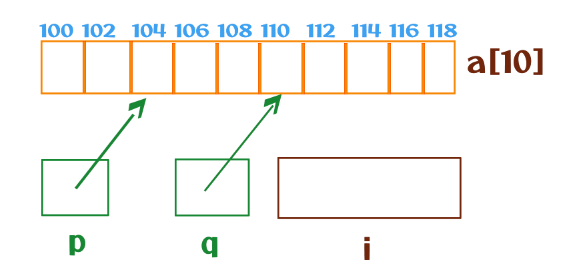
The fourth line of code p = p + 6 will have an output value of 116.
The pointer variable p will point to the starting address of the ninth element of the array, as shown in the image below.
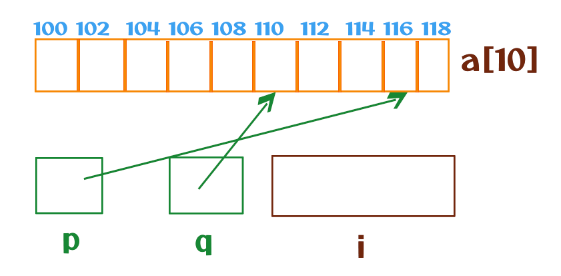
The fifth line of code q = p – 5 will have an output of 106 based on the formula of pointer arithmetic.
Now q will point to the fourth element of the array, as shown in the image below.
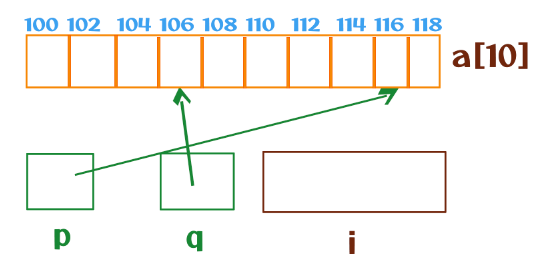
Similarly, the sixth line of code p = p- 6 will have an output of 104
Subtracting One Pointer from another
We will take the programming example in the image below for better understanding.
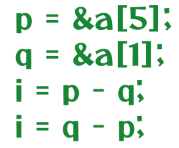
The visualization of the first and second lines of code is shown below.
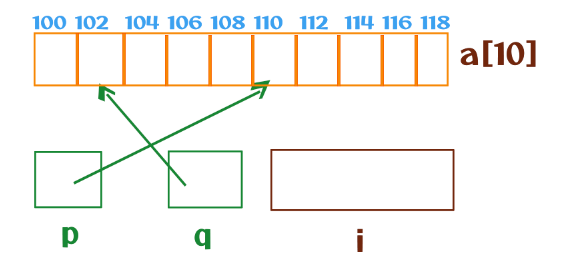
The third line of code i = p – q states that the address can be subtracted from one another.
The output of the above arithmetic is 4.
We will get the number of elements between those addresses.
The fourth line of code will produce an output of -4, which looks different, but we get that output.
Comparing Pointers
The image below is an example to understand the concept of pointer arithmetic.
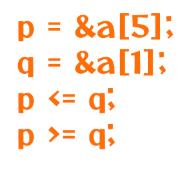
From the above example, the value stored in p is 110, and the value stored in q is 102.
The third line states that 110 <= 102, but the comparison is false, so 0 will be the output of the expression.
The fourth line states that 110 <= 102, the comparison is true so that one will be the output of the expression.