Type Conversion and Type Casting in Python
In this class, we discuss type conversion and type casting in python.
For Complete YouTube Video: Click Here
Type Conversion
Before going to discuss Type conversion. We should have an idea about operators. Please click here.
Let’s take an example and understand type conversion.
a=1
b=5.0
c= a+b
print(c)
From the above program, a is an integer type. b is a floating type.
c = a+b automatically c is assigned with the floating-point data type.
Float is of the higher type so this type of conversion happens implicitly by python.
Take one more example.
a=1
b=5+2j
c=a+b
a is of type int, and b is of type complex.
int will automatically convert to complex. ie 1+0j.
So c will be a complex type.
Take one more example where type conversion is not possible.
a=1
b=”25″
c=a+b
Is it possible to do addition on strings and integers?
No.
Here type conversion is not done.
Typecasting comes here.
Type Casting
Explicitly doing conversion is called type casting.
Example:
a=1
b=”25″
b= int(b)
c= a+b
print(c)
In the above program, we are converting the b value from string to int data type.
We use the function int to do this typecasting.
One more example of typecasting.
a=1
b=25.8
b= int(b)
c= a+b
print(c)
We are converting float to integer data type.
The integer part will only be considered here.
b will have the value of 25. The floating part is removed.
The output is 26.
List of Type Casting functions.
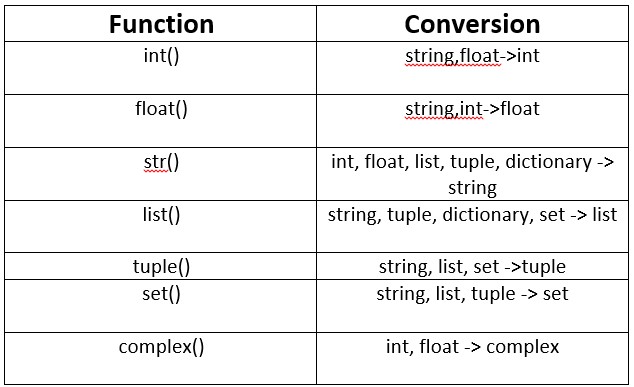