Memory Allocation to Functions and Garbage Collection in Python
In this class, we discuss Memory Allocation to Functions and Garbage Collection in Python.
For Complete YouTube Video: Click Here
Table of Contents
Memory Allocation to Functions
The reader should have prior knowledge of how memory is allocated to variables in python. Click here.
The memory allocation concept is very important to understand recursive function execution.
The discussion about recursive function is made in our next classes.
Python assigns some set of space to execute a program. The list is given in the below diagram.
Code space: The high-level code is converted to machine-level instructions, and the converted instructions are placed in RAM.
Global space: The programs global variables are given memory in the global space given to the program.
Heap space: Objects in the program are assigned memory in heap space.
Stack space: Function variables are provided memory in stack space.
Let’s take an example and understand how the memory is allocated during the execution of a program.
def f(x):
z=x+1
print(z)
def f1(y):
k=y+2
print(k)
a=1
f(a)
f1(a)
print(a)
In the above program, we have two functions, f and f1.
The program starts execution from line a=1.
What happens when the line a=1 executed?
In a =1. 1 is an integer object. This integer object is assigned memory in heap space.
This a=1 is a global variable. Therefore, the reference of the variable is maintained in global space.
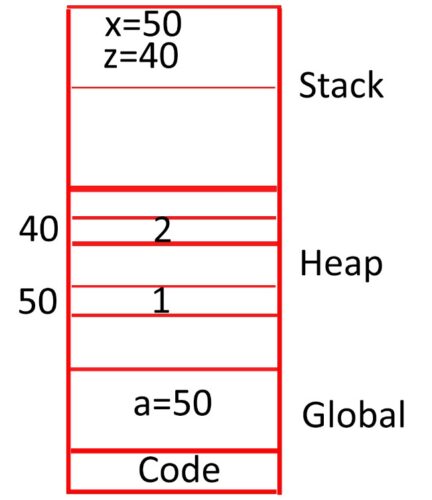
In our example, integer object one is assigned memory at location 50. shown in the above memory diagram.
In the memory diagram shown above. It was mentioned a=50 in global space. This a= 50 means a is referencing to memory location 50.
After execution of line a=1, the next line is f(a). function f is called.
What happens in the memory after calling the function f.
To execute the function, f. python will assign some memory in the stack. The stack memory is used to maintain the local variables of the function.
Function f had two variables, x and z. x is now a reference to memory location 50.
Because variable a is passed as an argument to the function. These basics are explained in the function concept. Click here.
Function f assigned value 2 for variable z. value two is set a memory location 40.
Variable z has maintained a reference to memory location 40.
After execution of function f. the memory assigned to the function is deleted.
Now the stack is empty. But still, object 2 is present in memory location 40. and no variable is referencing the integer object 2.
Garbage collection comes into the picture at this stage.
Garbage Collection
The garbage collection program will check the objects that are no more in use.
The garbage collection program identifies the objects, not in use and removes them from the heap.
In our example, integer object two is not referenced by any variable after completing the function.
After removing the integer object, we can use that memory for another object.
The need for garbage collection is to remove the unused objects from memory.
Now the function f1 is called. Memory for function f1 is assigned in the stack space.
Variables y and k maintain reference now. And after completion of the function, f1. The memory allocated will be removed.
Take one more example and understand the concept better.
def f(x):
z=x+b
print(z)
a=1
b=5
f(a)
In the above program, variable a and variable b are global variables.
Variable x and variable z are local variables to the function f.
In the function, f. variable b is used.
First, the variable is searched in function local variables memory.
Suppose the variable is not found in local memory. Then function checks in global variable memory.