More on scanf() Function
For Complete YouTube Video: Click Here
In this class, we will discuss More on scanf() function.
We consider the same example that we have considered in the Understanding scanf() function.
int main() { int i, j; float x, y, z; scanf("%d%d%f%f", &i, &j, &x, &y); printf("The values of i, j, x, y are:%d %d %f %f", i, j, x, y); scanf("%f", &z); printf("The value of z is %f", z); }
What are we going to understand in this class?
We will understand how the compiler will read the values of the scanf() function.
This class is fundamental because while reading the values, the compiler produces an unpredictable result if any mismatch happens.
scanf() Function
Let’s consider the first scanf() function of the program.
scanf(“%d%d%f%f”, &i, &j, &x, &y);
The image below is the way the user has provided the inputs.
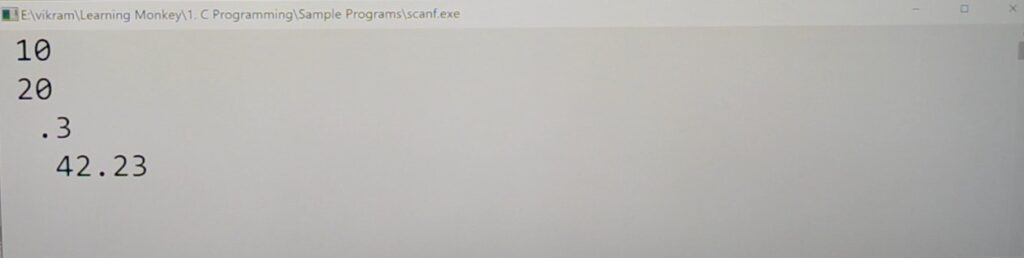
function
The user has given space in the above image and given input for the first variable, “i = 10”.
Next, he pressed an enter and space to provide the second input for the second variable, “j = 20”.
Similarly, the value of the third variable, “x = 0.3”, is provided after pressing an enter and two spaces.
The fourth variable, “y = 42.23”, pressed an enter and three spaces.
How compiler scans the values?
Let’s visualize the input given by the user more clearly.
For better understanding, consider a dash as space or enter.
The below is the visualization.
_ 10 _ _ 20 _ _ _ 0.3 _ _ _ _ 42.23 _
Now we will understand how the compiler scans the input.
The compiler first scans a space.
The compiler ignores the space.
Next, it scans 1 of the input 10.
Now the compiler will consider all the digits as the first input until it scans a space.
The compiler will ignore all the spaces after the last digit of the first input.
After scanning the first digit of the second input, the compiler will consider all the following digits as the second input.
The third conversion specifier in the format string is %f.
The compiler will scan for floating-point number.
The compiler identifies the first digit 0 and then scans a decimal point.
The digits before the decimal point are an integral part of a floating-point number.
After scanning a decimal point, the compiler will check for digits.
The compiler considers all the digits after the decimal point as the fractional part of the floating-point input.
Similarly, the compiler will scan the fourth input.
After entering the fourth input, the user will press enter, stating that the user provided all the information.
Now the compiler will execute the following lines of the program.
The second scanf() function will also get executed.
Mismatch in scanf() Function scanning
The image below is the input provided by a user.

In the above image, the user has given five inputs.
After providing five inputs, the user pressed enter.
Now the computer will not stop its execution for the second scanf() function.
The user’s fifth value is considered the value for the next scanf() function.
The image below is the output of the program.
