for Loop Pass and Nested Loop in Python
In this class, we discuss for loop pass and nested loop in python.
For Complete YouTube Video: Click Here
for Loop in Python
Before going into the concept of for loop, we should know the range function in python. Click here.
Let’s take examples and understand for loop in depth.
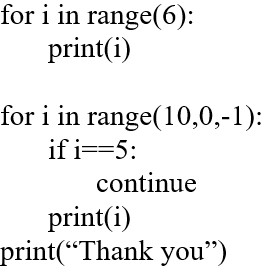
From the above program.
range(6) will generate a sequence of numbers from 0 to 5.
for loop will take the numbers one by one from the sequence.
Here i is the variable used in for loop. i will take values one by one. i.e. 0,1,2,3,4,5.
In the while loop, which we discussed in our previous class, there is some conditional check.
If the condition is True, then the loop executes; otherwise, it come out of the loop.
In for loop, we don’t have such conditions. Take the values from the sequence and go to execute loop statements each time with the value chosen.
The second program is to display numbers from ten to one. But five should not be displayed.
This example was already done using a while loop in our last class.
The 2nd program is the same code using for loop.
for loop with else
Whenever the loop completes its execution, it goes to block else.
Program:
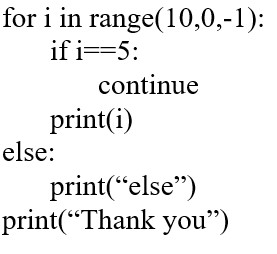
The output displayed by the above program
10
9
8
7
6
4
3
2
1
else
Thank you.
We are not giving an example on the break statement.
Hoping that you already watched our while loop class explained before.
In the same way, we use the break statement to come out of the loop.
Pass
If there is nothing to execute inside the loop. We use a pass statement.
Python compiler will understand by looking at pass statement. It decides there is nothing to execute in a loop.
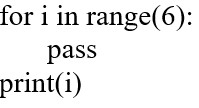
The above program displays the output.
5
why is i 5?
The above program for loop executed. means i value takes values 0,1,2,3,4,5. end of loop i =5.
for loop executed, but there is nothing to do in the loop. That’s the meaning of pass.
Don’t think that, for loop not executed.
We can use pass statement in if condition, functions, and class. We discuss them later.
Example using pass in if condition.
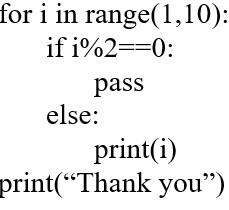
The above program displays odd numbers from 1 to 10.
To implement the logic, we are using the pass in if statement.
The condition in if statement checks i is even or odd. If i is even, the condition is True.
If the condition is True, do nothing. For that reason, we use a pass statement.
i%2 value will be zero if i is an even number.
Nested Loop
A loop within loop we call nested loop.
The same way if within if we call nested if. We discussed it previously.
It is essential to understand nested loops.
Most of the coding depends on Nested loops.
Let’s take an example and understand how the nested loop executes.
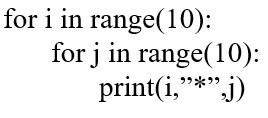
The above program has a loop inside a loop.
We call loop with i variable as the outer loop.
Loop with j variable as the inner loop.
The outer loop takes the values 0,1,2…9. Means loop executes ten times.
In the body of the outer loop, we have one more loop. We call the inner loop.
This inner loop also executes ten times with the values 0,1,2,…9.
The inner loop body has a print statement that displays i value, multiplication symbol *, and j value.
How this code executes?
With i value 0. i.e. outer loop value zero. Inner loop executes ten times i.e j takes 0,1,2,…9.
With i value 1. i.e. outer loop value one. Inner loop executes ten times. i.e j takes 0,1,2,…9.
The outer loop repeats till i =9. i.e. outer loop takes all the values.
The output displayed by the program is shown below.
0 * 0
0 * 1
0 * 2
.
.
.1 * 0
1 * 1
.
.
.
9 * 9