C Programming Practice 1 on Arithmetic Operators
For Complete YouTube Video: Click Here
In this class, we will do C Programming Practice 1 on Arithmetic Operators.
We have covered Arithmetic, Assignment, Increment-Decrement, Bitwise, Shift, Ternary, Relational, and Logical Operators.
Here we will solve some C Programming Practice on Arithmetic Operators.
Table of Contents
Programming Practice
Below are the two programs to practice on Arithmetic Operators.
Program 1:
int main() { int a = 2, b = 3, c = 7, d = 1, e = 12, f = 3, g = 20, h; h = a + b * c - d * e / f + g; printf("%d", h); return 0; }
Program 2:
int main() { printf("%d", 6*2/( 2+1 * 2/3 +6) +8 * (8/4)); return 0; }
For a better understanding of the programs, consider the Operator Precedence And Associativity Table image given below.
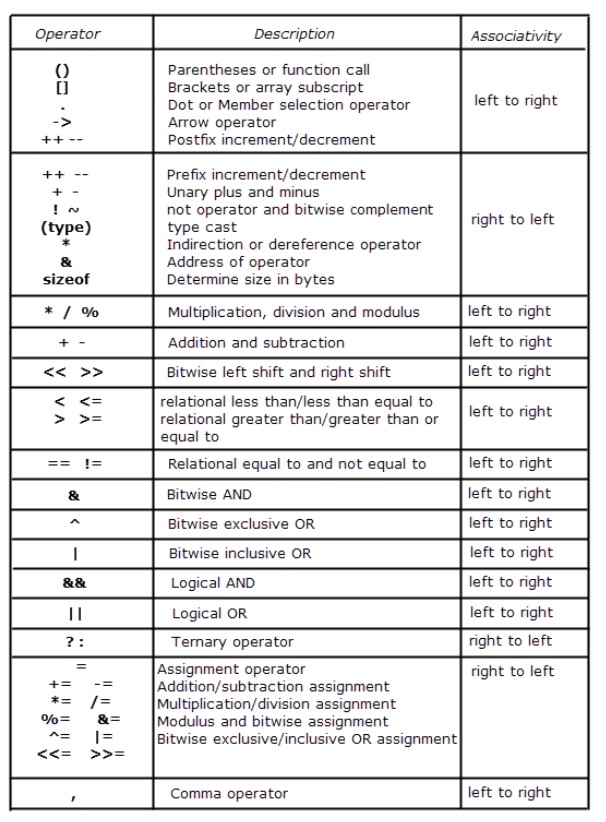
Program 1
Program 1 is on Arithmetic operators
int main() { int a = 2, b = 3, c = 7, d = 1, e = 12, f = 3, g = 20, h; h = a + b * c - d * e / f + g; printf("%d", h); return 0; }
In program1, we have eight variables from a-h.
Let us substitute the values of the variables in the above expression.
h = 2 + 3 * 7 – 1 * 12 / 3 + 20.
In the above expression, all are arithmetic operators.
From the operator precedence and associativity table, the multiplicative operators have the highest priority over additive operators.
The multiplicative operators have left to right associativity.
The compiler will execute the expressions enclosed in the parentheses from left to right.
h = 2 + (3 * 7) – ((1 * 12) / 3) + 20.
h = 2 + 21 – 4 + 20.
Now the above expression has additive operators.
As all the operators have the same priority, the expression will be executed from left to right.
h = (((2 + 21) – 4)+ 20)).
h = 39.
Program 2
Program 2 is on Arithmetic operators
int main() { printf("%d", 6*2/( 2+1 * 2/3 +6) +8 * (8/4)); return 0; }
In the above program, the expression to be evaluated is as shown below.
6*2/( 2+1 * 2/3 +6) +8 * (8/4)
From the operator precedence and associativity table, it is evident that parentheses have the highest priority.
The parentheses have associativity of left to right.
So, ( 2+1 * 2/3 +6) will be executed first and then 8/4.
Within the parentheses, the multiplicative operators will be executed first and the additive operators.
6* 2/8+8*2
In the above expression, all the multiplicative operators will be executed from left to right.
1 + 16 is equal to 17.
The final output is 17.