Square and Triangle Patterns in Python
In this class, we do practice on square and triangle patterns in python.
For Complete YouTube Video: Click Here
Square Pattern
Before practice, you should have some basic knowledge of nested loops. Click here.
Here we will concentrate on how to do coding for a given problem. These simple programs help you in solving the complex programs we discuss in subsequent classes.
Q1) Write a program to display the square pattern shown below.
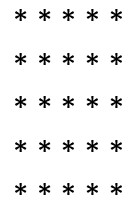
The program has to take input about the side of the square. It should be an integer input.
For example, if the side of the square value is 5. we have to display a 5X5 square shown above.
Suppose the input value is six. Display a 6X6 square.
Logic:
Suppose the input is given as 5. In the first line, we have to display 5-star symbols with space between them.
The second line, display five stars.
In the same way, we have to display five lines.
Previously we discussed when to uses loops.
Something to do repeatedly we use loops.
To display five stars, we use a loop.
To repeat the above loop five times. We need a nested loop.
The program to display the square pattern is shown below.
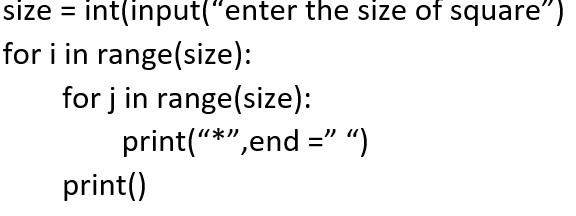
From the above program, the loop statement with i variable executes five times.
Inside the loop, we have written another loop that executes five times.
The inner loop is displaying one line with five stars.
To display stars in the same line, use print(“*”,end=” “)
For each outer loop, the inner loop will display a line of five stars.
Triangle Pattern
Q2) Write a program to display the triangle pattern given below.
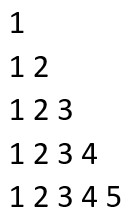
Take the input from the user. If the input is given 5. Display the above triangle pattern.
First-line display 1.
The second line display 1 2.
Similarly, in each line, increase the numbers to be displayed by 1.
We can analyze that this program needs a nested loop.
The program is given below.
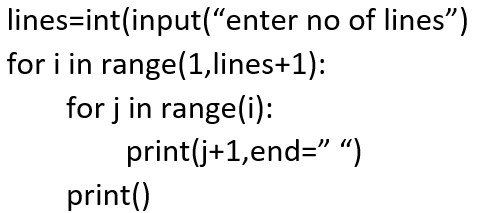
The outer for loop will execute five times from the above program if the line variable value is 5.
Each time it went into the loop, the inner loop executes for i times. i is a variable from the outer loop.
The first iteration of the outer loop i value is 1. The inner loop executes one time and displays 1.
The second iteration of the outer loop i value is 2. The inner loop executes two times and displays 1 2.
The outer loop executes five times.
Related links: