Mutable vs Immutable Object in Python
In this class, We discuss mutable vs immutable object in python.
For Complete YouTube Video: Click Here
Table of Contents
Immutable Object
The reader should have prior knowledge of Memory allocation to variables. Click here.
Knowledge of string and list data types is also needed. Click here.
We did not have any discussion about the object in our previous classes.
For now, remember, in python, everything is an object.
Let’s take an example and understand the mutable and immutable object.
x=20
y=x
From our previous knowledge of how memory is allocated to variables.
x=20. 20 is an integer object. 20 is assigned a memory location 50.
y=x means y is referencing the same memory location variable x is referencing. I.e., memory location 50.
An immutable object means an object which is not allowed to change its contents. We call immutable objects.
From the above example, an integer is an immutable object.
20 is not allowed to change its content.
What happens if we try to change the content of the object?
Take x = x+1
The above statement is trying to modify the content of an integer object.
It will create a new object 21, and this new object 21 is given reference to variable x.
It is shown in the diagram below.
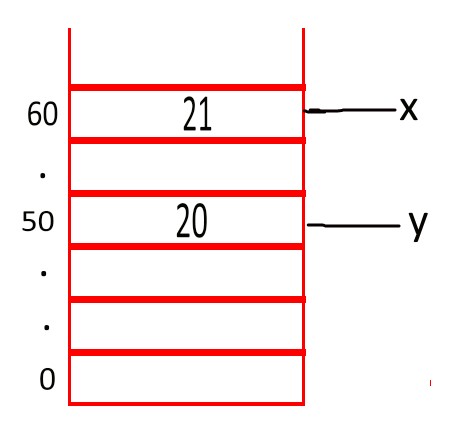
Now x is referencing memory location 60. which contains object 21.
y is referencing memory location 50. which contains object 20.
Object 20 is not modified. That is immutable object means.
Mutable Object
To understand the concept of a mutable object. Please watch how memory is allocated to the list. Click here.
The list is an example of a Mutable object.
Let’s take an example and understand the mutable object.
x=[1,2,5]
y=x
x is a list object. how memory allocated is shown below.
x=[50,60,70]
integer one is assigned a memory location 50. That address is saved in the list.
The same way integer two is assigned a memory location 60. That address is saved in the list. and so on.
x and y are referencing the same list.
The list is allowed to change its contents.
x.append(6). we have assigned a new value 6 to list x.
x=[50,60,70,80]. the new element is assigned a memory location 80. and saved in the list.
Now both x and y are pointing to the same list. The modification did not create a new list.
The above example, i.e., the list example, is the meaning of the mutable object.
The list of immutable objects.
- boolean
- int
- float
- complex
- tuple
- string
The list of mutable objects.
- list
- set
Practical code
x=10
y=x
print(id(x))
print(id(y))
Output: 8291408, 8291408 Both pointing same location
Modify x
x=x+1
print(id(x))
print(id(y))
Output: 8291424, 8291408 Both pointing to different location
a=[1,2,5]
b=a
print(id(a))
print(id(b))
Output: 3038982604, 3038982604 Both pointing same location.
a.append(6)
print(id(a))
print(id(b))
Output: 3038982604, 3038982604 Both pointing same location after modification.