Shift Operators in C Left Shift and Right Shift
For Complete YouTube Video: Click Here
We will try to understand the Shift Operators in C Left Shift and Right Shift in this class.
Table of Contents
Shift Operators in C
Shift operators are the types of bitwise operators.
Some of the bitwise operators are explained in our previous class.
As the shift operators are bitwise operators, the operations are done on the bits of the values of the variables.
The image below shows different types of bitwise operators.
The shift operators in C are part of bitwise operators they are divided in left shift and right shift operators.
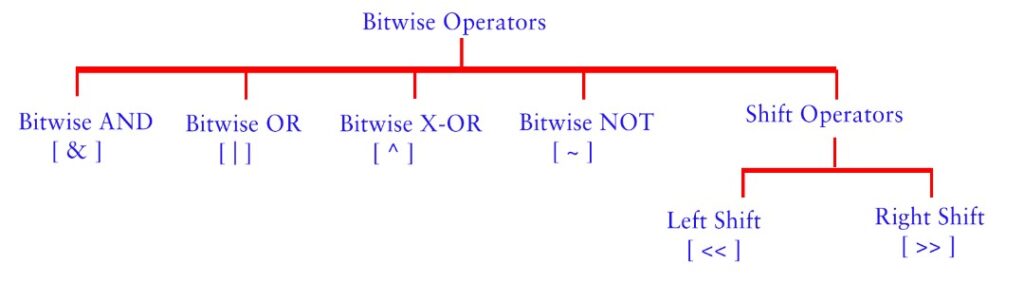
The program below illustrates the concept of shift operation.
int main() { int a = 12; printf("Left Shift a<<1 is %d\n", a<<1); printf("Right Shift a>>1 is %d\n", a>>1); }
Types of Shift Operators in C
The shift operators are of two types
- Left Shift Operator [ << ]
- Right Shift Operator [ >> ]
Left Shift Operators [ << ] in C
Left shift operator shifts all bits towards the left by a certain number of specified bits.
The first printf of the above program uses the left shift operator.
In the above program, a is a variable with 12 stored in it.
The binary value of 12 is 00001100 in an 8-bit representation.
For decimal to binary conversions use these tools.
“a” << 1 states that the bits stored in a are shifted to the left.
The binary value of the variable “a” after shifting is 00011000.
The benefit of left shifting by 1 multiplies the binary value by 2.
The decimal equivalent of the binary value after shifting is 24.
Similarly, the expression a << 2 will multiply a with 2^2 times. [12 * 2^2 = 48]
The value in a will be 48.
If we observe the printf function, the bits will shift, and the printf will print [24] the value after moving.
If we print the value “a” in another print statement, it will publish 12, not 24.
Because in the above printf function, the bits after shifting are assigned to a variable.
The above point is essential to understand.
To understand the above point clearly try to execute the program below
int main() { int a = 12; printf("Left Shift a>>1 is %d\n", a>>1); printf("Left Shift a>>1 is %d\n", a); }
The output of the above program is as shown in the image below.

In the above output the first printf has shifted and printed but not assigned the shifted value to a variable.
Consider the program below.
int main() { int a = 12; printf("Left Shift a>>1 is %d\n", a = a>>1); printf("Left Shift a>>1 is %d\n", a); }
The output of the above program is shown in the image below.

The value after shifting is assigned to the variable a.
The value of a is now changed.
Right Shift Operators [ >> ] in C
The Right shift operator shifts all bits towards the right by a certain number of specified bits.
The second printf of the above program uses the right shift operator.
“a” >> 1 states that the bits stored in a are shifted to the right.
The binary value of the variable “a” after shifting is 00000110.
The benefit of left shifting by 1 divides the binary value by 2.
The decimal equivalent of the binary value after shifting is 6.
Similarly, the expression a << 2 will divide a with 2^2 times. [12 / 2^2 = 3]
The value in a will be 3.