Pyramid Patterns in Python
In this class, we do practice pyramid patterns in python.
For Complete YouTube Video: Click Here
Pyramid Patterns
For better logic development, first complete square and triangle patterns. Click here.
All the examples from this course will help you in solving complex programs in the placement course.
Q1) Write a program to display the pyramid pattern given below.
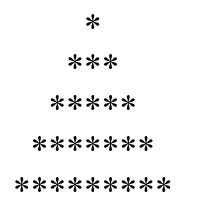
The program has to take the input about the number of lines.
Construct a pyramid with a height equal to the number of lines.
The first line with one star.
The Second line consists of three stars.
The third line consists of five stars. So on continue till the number of lines given.
Logic
From the above diagram, we observe that we have given some spaces and a star symbol in the first line.
In the second line, we displayed some spaces and then stars.
Reduce the number of spaces to be displayed and increase star symbols. As we move down.
Here we need a nested loop. Because displaying a line need a loop.
We have to display multiple lines, so a loop within a loop is required.
The program is given below.
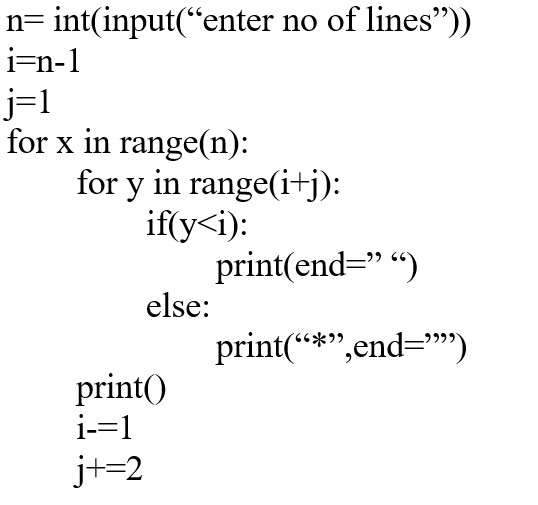
From the above program, we have to observe the main logic lies with i and j variables.
Variable i is used for spaces. And variable j is used to represent the number of star symbols.
Initially, variable i considered value as n-1 because n-1 spaces given.
Variable j value is taken value 1. because a one-star symbol has to be displayed.
After each iteration reducing the variable, i value by 1.
Increase the variable j value by 2.
The inner loop is executing i+j times to display spaces and star symbols using if conditional statements.
Try to analyze the code for better understanding. That’s the best practice.
Q2) Write a program to display the inverse pyramid given below.
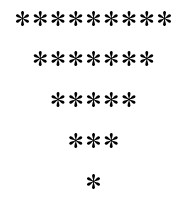
Logic
Precisely similar to Q1.
Just display in reverse.
First-line consists of zero spaces and (2*number of lines-1) star symbols.
Second-line one space and reduce star symbols by 2.
Based on this logic Initialize i = 2*n -1.
j=0
The remaining logic was the same as our above program.
Check the code given below and analyze it for better understanding.
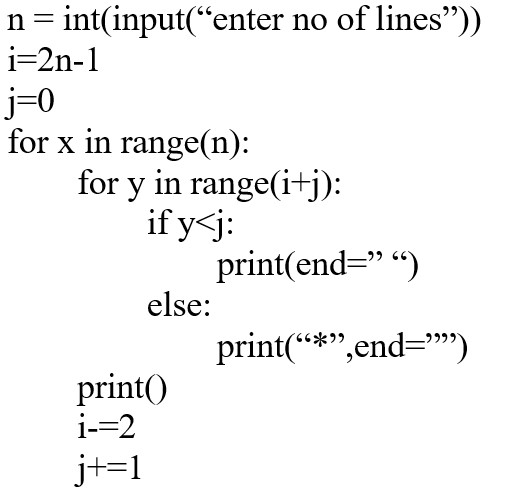