Understanding scanf() Function
For Complete YouTube Video: Click Here
In this class, we will try to have Understanding scanf() Function.
The scanf() is a formatted input function.
We already discussed the formatted output printf() function, conversion specifier, and escape sequence.
Please go through the above links before proceeding further.
scanf() Function
We will try to understand the concept of scanf() with a programming example given below.
int main() { int i, j; float x, y, z; scanf("%d%d%f%f", &i, &j, &x, &y); printf("The values of i, j, x, y are:%d %d %f %f", i, j, x, y); scanf("%f", &z); printf("The value of z is %f", z); }
The printf() function will print the content of the format string.
Similarly, the scanf() function will also read the content specified in the format string.
The format string of the scanf() may have the characters and conversion specifiers, but it is not a good practice to have the charters or special characters.
So far, in our previous programming practices, we have seen that int a = 10, b = 20. All the variables are assigned within the program declaration.
On the other hand, the user of the program can assign the values to the variables.
Let us analyze every line of code in the above program.
The first two lines of the code declare the two integer variable i, j and three floating-point variables x, y, and z.
The image below shows the allocation of the memory to the variables by the compiler.
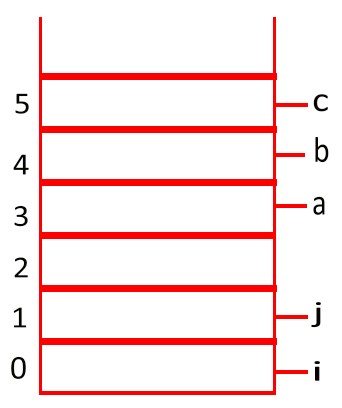
scanf() Function
The third line of code is the use of the scanf() function, as shown below.
scanf(“%d%d%f%f”, &i, &j, &x, &y);
Here we used four conversion specifiers in the format string.
The variables after the format string are attached with the ampersand & symbol.
The printf() function does not used & symbol.
In scanf(), we have to use the & symbol because attaching & to the variable indicates the memory’s address allocated to the variable by the compiler.
In executing the program, whenever a scanf() function is there in the program, the compiler will wait for the user to provide the values.
How will the compiler differentiate one input from other?
The compiler will differentiate one input from other by providing space or by pressing an enter.
For example, the user has provided the values as 10 -20 0.3 40.24.
Ten will be stored in the variable “i”, -20 will be held in j, 0.5 in variable x, and 40.24 in variable y.
After providing all the values by pressing enter, the compile will execute the following line of code.
The enter pressed after providing all the values will be considered in the program’s next scanf() function.
Now the compiler will execute the printf() function.
The output of the printf() function is as shown below.
The values of I, j, x, y are 10 -20 0.5 40.24.
Now the compiler will stop its execution for the user to provide the value of z.
After providing the value and pressing enter, the compiler will execute the printf() function.