No of Digits in a Number Swap Digits Using Loops
In this class, we practice no of digits in a number and swap digits using loops.
For Complete YouTube Video: Click Here
Digits in a Number
The examples which we discuss will help you a lot in placement interviews and improving coding skills. Please check our placement course for more standards and practice tests.
We must have some basic knowledge of loops to do these examples. Click here.
We give logic hints and code. Try to understand logic hints and write the code on your own.
Q1) Write a program to check the given number is divisible by the number of digits in that number.
Let’s take an example and understand the logic to write the program.
12345 take this number. How many digits are there in the given number?
Five digits are there in the number.
Is 12345 divisible by 5? Yes.
We have to display yes given number is divisible by the number of digits in the number.
Otherwise, we have to display it’s not divisible.
Logic:
12345/10 = 1234
1234/10 = 123
123/10 =12
12/10=1
1/10 =0
To identify the number of digits in a number. Divide the number until it became zero.
We use the loop to divide the number to zero.
Each time we go into loop increment count by 1. the count variable used to count no of digits.
We use floor division because we consider only integer part.
Check the while loop from the program given below.
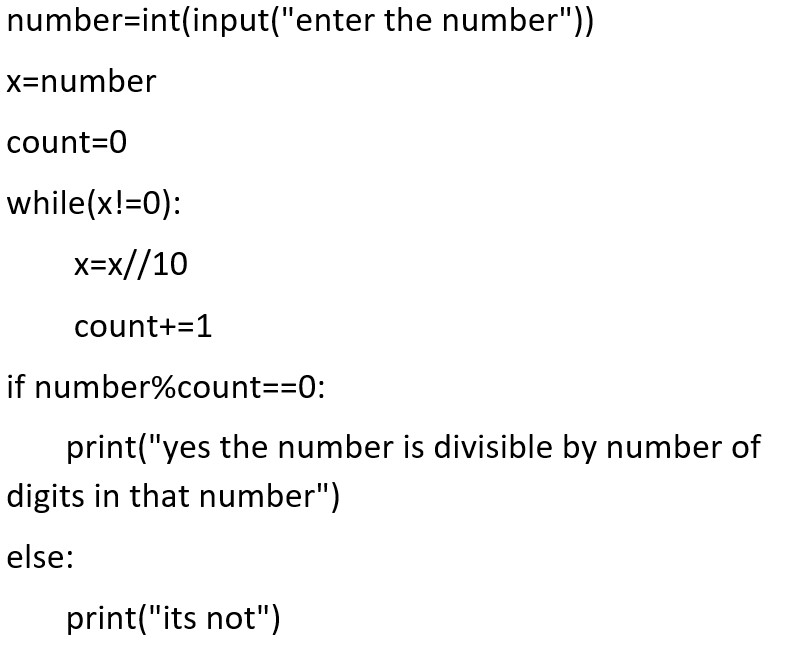
Swap
Q2) Write a program to swap the first and last digits in a given number.
Let’s take an example and understand the question.
12345 is a number provided.
The output should be 52341. here we swapped the first digit and last digit.
Logic:
Here we have to identify 1st digit, last digit, and the number of digits.
From the first program, we know how to identify the number of digits.
From the given example, the first digit is 5.
the last digit is 1.
We do 10 power count-1 * first digit. Here count is the number of digits. The value is 50000.
Same ass 10 power count-1 * last digit. The value is 10000
Now 12345 + 50000 = 62345
62345 – 10000 = 52345
52345 + 1 = 52346
52346 – 5 = 52341—-> output
We follow the above sequence of arithmetic operations to bring the required output.
We use the modulus operator to identify the first digit and last digit because the modulus will give the reminder value.
Check the below program for better coding understanding.
